- With Visual Studio open, left-click FILE in the menubar, mouse over New in the menu, and left-click Project in the submenu. This pops up the New Project dialog. Left-click Visual C under Templates in the left-hand pane, and left-click Win32 Console Application in the center pane. Change the name of the project by left-clicking the box next to.
- Create a.NET console app project named 'HelloWorld'. Start Visual Studio 2019. On the start page, choose Create a new project. On the Create a new project page, enter console in the search box. Next, choose C# or Visual Basic from the language list, and then choose All platforms from the platform list.
- Run Visual Studio and select File - New - Project in the application menu. In the New Project window, select the Visual C template - Windows and in the next menu select Empty Project. Let's name this project 'FirstApplication'. Create a folder for your projects in your Dropbox folder, for example, 'cpp/' (as in Plus Plus).
A Simple Class Program Example This example will show you how to:
1.Start Microsoft Visual C++/Studio .NET. 2.From the File menu, select New and then select Project. The New Project dialog box appears. |
3.Set the Project Type to CLR Projects.
4.Set the Templates to CLR Console Application.
5.Type Animals in the Name text box.
If you don't see the Console App (.NET Core) project template, you can get it by adding the.NET Core cross-platform development workload. Option 1: Use the New Project dialog box. Choose the Open Visual Studio Installer link in the left pane of the New Project dialog box. The Visual Studio Installer launches.
6.Choose appropriate location for the new project. Click OK.
7.In Solution Explorer, double-click the Animals.cpp file in the Source Files folder.
8.Immediately under the usingnamespaceSystem; line, add the following class definition:
refclass Animal
{
int legs;
String^ strName;
};
To declare a class in C++, you use the keyword class followed by a name for the class, such as Animal in this example, and then you list all the class’s member variables, functions, and methods between an opening brace ({) and a closing brace (}). So far, you have created an Animal class with an int variable for the number of its legs and a String variable for its name. As it stands, no other program or class will be able to access these variables. The members of a class, data and methods, are private by default and can only be accessed by methods of the class itself. C++ provides three access modifiers: public, private, and protected, to specify the visibility of the various members of the class.
9.Add the keyword public followed by a colon (:) on a new line between the opening brace and the first variable declaration, as shown here:
refclass Animal
{
public:
int legs;
String^ strName;
};
Declaring the variables after the keyword public makes both of them accessible. However, it is not usually a good idea to allow other classes and parts of your program access to the variables of a class. As discussed earlier in the section on encapsulation, it’s better to keep the implementation details of a class hidden from users of that class and to control the access to the class’s data through functions. In this example, the keyword private will be used to prevent direct access to the String variable of the class. The int variable legs will be left with public access, simply to show how it can then be directly accessed by the main program.
10.Add the keyword private followed by a colon (:) between the first int variable and the second String variable, as shown here:
refclass Animal
{
public:
int legs;
private:
String^ strName;
};
To provide access to the private String variable, public accessor functions and methods need to be added to the class to allow other functions to manipulate its value.
11.After the declaration of the int variable and before the private access modifier, add the following method declarations or implementation lines:
void SetName(String^ name) { strName = strName->Copy(name); }
String^ GetName() { return strName; }
Because these methods are small functions, it’s easiest to declare and implement them as in-line functions. The Animal class is now complete. The syntax of the declaration is:
ref class classname
{

Access control keywords (public, private or protected)
The declaration of class variables and methods
}
More complete syntax is given below.
class_access ref class name modifier : inherit_access base_type { };
Example:
refclass MyClass2 {
public:
int i;
};
refclass MyClass2 : public MyClass::MyInterface {
public:
virtualvoid f() { System::Console::WriteLine('testing 1..2..3'); }
You have probably noticed the ref keyword. This keyword is one of the new C++ keyword (Managed Extensions for C++ uses __gc) that simplifies the interaction with .NET Framework components. By placing ref in front of the class keyword, the class becomes a managed class. When the object is instantiated, it will be created on the common language runtime (CLR) heap and the gcnew (instead of new) operator will return the memory address of this object. The lifetime of an object instantiated from the class will be managed by the .NET Framework’s garbage collector. When the object falls out of scope, the memory used by the object will be garbage-collected and no explicit calls to delete (such as delete keyword) will have to be made. ref classes are known as reference types because the variable does not actually contain the object but a pointer to the memory where the object is. However, there are performance issues to consider when using reference types. The memory has to be allocated from the managed heap, which could force a garbage collection to occur. In addition, reference types have to be accessed via their pointers, affecting both the size and speed of the compiled application. Because of these performance issues, the .NET Framework also supports value types. Value types are objects created on the stack. The variable contains the object itself rather than a pointer to the object. Hence, the variable doesn’t have to be dereferenced to manipulate the object, which of course improves performance. To declare a value type class, the value keyword should be used instead of the ref keyword. In this case, the variables would have been created on the stack. Instead of declaring pointers for this class and then creating the objects on the CLR heap by using the new operator, the objects would have been declared in the same way as the built-in C++ types and the member variables accessed by the dot operator (.) rather than via the dereferencing operator (->). Now that the Animal class has been constructed, it can be used by the program just as the program would use a built-in type.
12.In the main() function, delete the following line:
13.Declare and create two Animal objects in your main() function, as shown here:
Animal ^ lizard = gcnew Animal;
Animal ^ bird = gcnew Animal;
The keyword gcnew followed by the class of the object being created creates the object on the CLR heap rather than on the stack. The memory address of the created object is returned and stored in the pointer.
14.Use the member function SetName to assign the names Lizard and Bird to the respective lizard and bird objects, and set the legs variable for both objects as shown below.
lizard->SetName('Lizard');
lizard->legs = 4;
bird->SetName('Bird');
bird->legs = 2;
To access the member variables and functions, you have to dereference the pointer in one of two ways. You can use:
The dereferencing operator, an asterisk (*) followed by the dot notation, for example, (*lizard).legs.
You can also use the shorthand operator for indirect access, which is a minus sign and right angle bracket (->).
15.Having created a couple of Animal objects and assigned data to them, you are now going to display that data on the screen. Add the following lines:
Console::WriteLine('Animal object #1');
Console::Write('Name: ');
Console::WriteLine(lizard->GetName());
Console::Write('Legs: ');
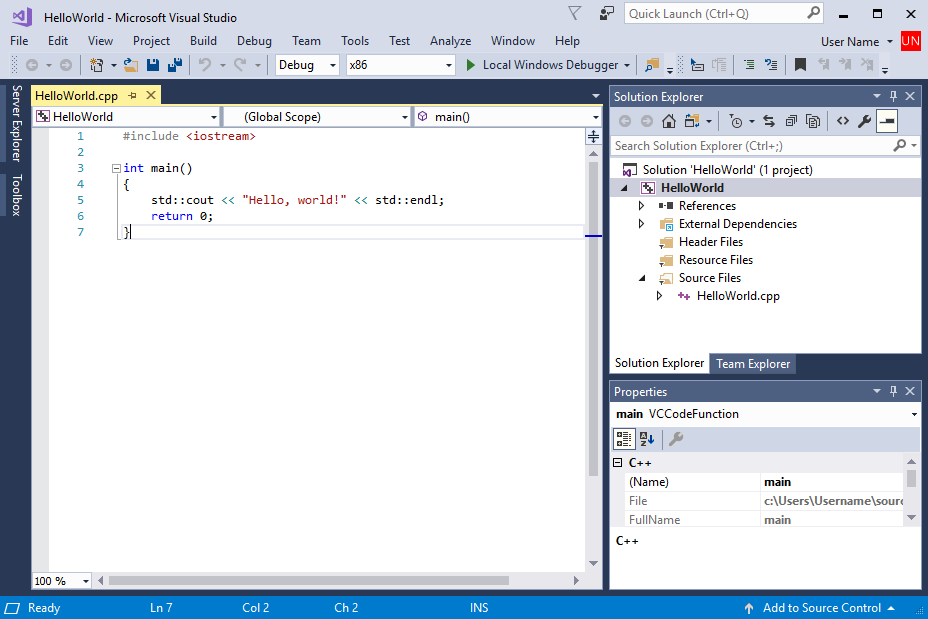
Console::WriteLine(lizard->legs);
Console::WriteLine();
Console::WriteLine('Animal object #2');
Console::Write('Name: ');
Console::WriteLine(bird->GetName());
Console::Write('Legs: ');
Console::WriteLine(bird->legs);
Console::WriteLine();
16.Build the application. Select Build Solution from the Build menu bar, or use the keyboard shortcut Ctrl+Shift+B.
In case you’ve had any problems putting the program together from the fragments in the preceding steps, the entire program is listed here:
// Animal.cpp : main project file.

#include'stdafx.h'
usingnamespace System;
refclass Animal
{
public:
int legs;
void SetName(String^ name) { strName = strName->Copy(name); }
String^ GetName() { return strName; }
private:
String^ strName;
};
int main(array<System::String ^> ^args)
{
Animal ^ lizard = gcnew Animal;
Animal ^ bird = gcnew Animal;
lizard->SetName('Lizard');
lizard->legs = 4;
bird->SetName('Bird');
bird->legs = 2;
Console::WriteLine('Animal object #1');
Console::Write('Name: ');
Console::WriteLine(lizard->GetName());
Console::Write('Legs: ');
Console::WriteLine(lizard->legs);
Console::WriteLine();
Console::WriteLine('Animal object #2');
Console::Write('Name: ');
Console::WriteLine(bird->GetName());
Console::Write('Legs: ');
Console::WriteLine(bird->legs);
Console::WriteLine();
return 0;
}
17.If the build was successful, run the application by selecting Start Without Debugging from the Debug menu, or use the keyboard shortcut Ctrl+F5.
The output is shown below.
Part 1 | Part 2 | Part 3 | Part 4 | Part 5 | Part 6 | Part 7 | Part 8
-->The usual starting point for a C++ programmer is a 'Hello, world!' application that runs on the command line. That's what you'll create in Visual Studio in this step.
Prerequisites
- Have Visual Studio with the Desktop development with C++ workload installed and running on your computer. If it's not installed yet, see Install C++ support in Visual Studio.
Create your app project
Visual Studio uses projects to organize the code for an app, and solutions to organize your projects. A project contains all the options, configurations, and rules used to build your apps. It manages the relationship between all the project's files and any external files. To create your app, first, you'll create a new project and solution.
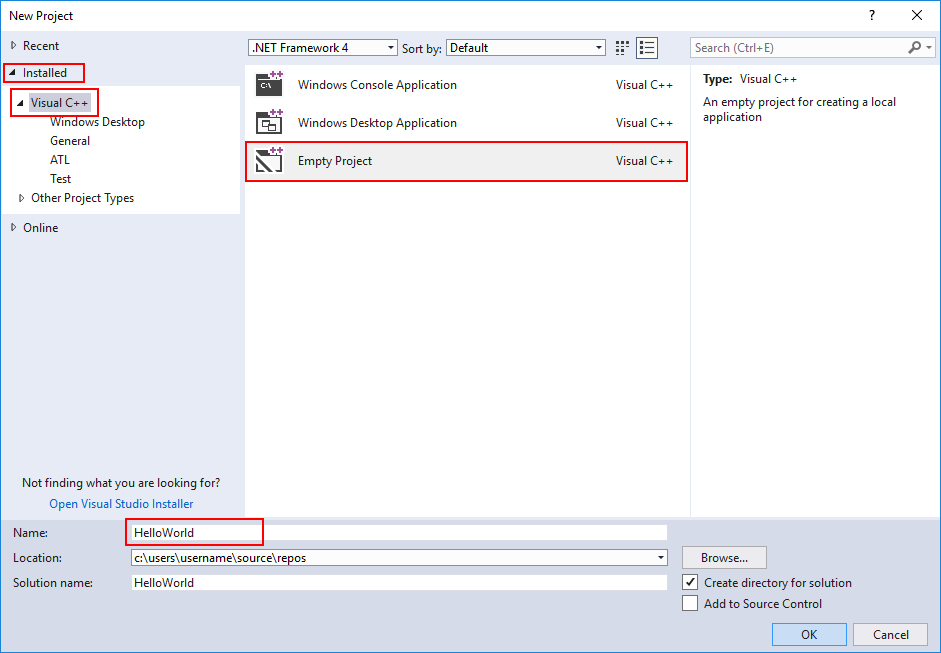
In Visual Studio, open the File menu and choose New > Project to open the Create a new Project dialog. Select the Console App template that has C++, Windows, and Console tags, and then choose Next.
In the Configure your new project dialog, enter HelloWorld in the Project name edit box. Choose Create to create the project.
Visual Studio creates a new project. It's ready for you to add and edit your source code. By default, the Console App template fills in your source code with a 'Hello World' app:
When the code looks like this in the editor, you're ready to go on to the next step and build your app.
In Visual Studio, open the File menu and choose New > Project to open the New Project dialog.
In the New Project dialog, select Installed > Visual C++ if it isn't selected already, and then choose the Empty Project template. In the Name field, enter HelloWorld. Choose OK to create the project.
Visual Studio creates a new, empty project. It's ready for you to specialize for the kind of app you want to create and to add your source code files. You'll do that next.
Make your project a console app
Visual Studio can create all kinds of apps and components for Windows and other platforms. The Empty Project template isn't specific about what kind of app it creates. A console app is one that runs in a console or command prompt window. To create one, you must tell Visual Studio to build your app to use the console subsystem.
In Visual Studio, open the Project menu and choose Properties to open the HelloWorld Property Pages dialog.
In the Property Pages dialog, select Configuration Properties > Linker > System, and then choose the edit box next to the Subsystem property. In the dropdown menu that appears, select Console (/SUBSYSTEM:CONSOLE). Choose OK to save your changes.
Visual Studio now knows to build your project to run in a console window. Next, you'll add a source code file and enter the code for your app.
Add a source code file
In Solution Explorer, select the HelloWorld project. On the menu bar, choose Project, Add New Item to open the Add New Item dialog.
In the Add New Item dialog, select Visual C++ under Installed if it isn't selected already. In the center pane, select C++ file (.cpp). Change the Name to HelloWorld.cpp. Choose Add to close the dialog and create the file.
Visual studio creates a new, empty source code file and opens it in an editor window, ready to enter your source code.
Add code to the source file
Copy this code into the HelloWorld.cpp editor window.
The code should look like this in the editor window:
When the code looks like this in the editor, you're ready to go on to the next step and build your app.
Next Steps
Troubleshooting guide
Come here for solutions to common issues when you create your first C++ project.
Create your app project: issues
The New Project dialog should show a Console App template that has C++, Windows, and Console tags. If you don't see it, there are two possible causes. It might be filtered out of the list, or it might not be installed. First, check the filter dropdowns at the top of the list of templates. Set them to C++, Windows, and Console. The C++ Console App template should appear; otherwise, the Desktop development with C++ workload isn't installed.
To install Desktop development with C++, you can run the installer right from the New Project dialog. Choose the Install more tools and features link at the bottom of the template list to start the installer. If the User Account Control dialog requests permissions, choose Yes. In the installer, make sure the Desktop development with C++ workload is checked. Then choose Modify to update your Visual Studio installation.
If another project with the same name already exists, choose another name for your project. Or, delete the existing project and try again. To delete an existing project, delete the solution folder (the folder that contains the helloworld.sln file) in File Explorer.
Go back.
If the New Project dialog doesn't show a Visual C++ entry under Installed, your copy of Visual Studio probably doesn't have the Desktop development with C++ workload installed. You can run the installer right from the New Project dialog. Choose the Open Visual Studio Installer link to start the installer again. If the User Account Control dialog requests permissions, choose Yes. Update the installer if necessary. In the installer, make sure the Desktop development with C++ workload is checked, and choose OK to update your Visual Studio installation.
If another project with the same name already exists, choose another name for your project. Or, delete the existing project and try again. To delete an existing project, delete the solution folder (the folder that contains the helloworld.sln file) in File Explorer.
Visual Studio Console Application C++
Go back.
Make your project a console app: issues
If you don't see Linker listed under Configuration Properties, choose Cancel to close the Property Pages dialog. Make sure that the HelloWorld project is selected in Solution Explorer before you try again. Don't select the HelloWorld solution, or another item, in Solution Explorer.
The dropdown control doesn't appear in the SubSystem property edit box until you select the property. Click in the edit box to select it. Or, you can press Tab to cycle through the dialog controls until SubSystem is highlighted. Choose the dropdown control or press Alt+Down to open it.
Add a source code file: issues
It's okay if you give the source code file a different name. However, don't add more than one file that contains the same code to your project.
If you added the wrong file type to your project, such as a header file, delete it and try again. To delete the file, select it in Solution Explorer. Then press the Delete key.
Visual Studio Console Application C++
Go back.
Add code to the source file: issues
If you accidentally closed the source code file editor window, you can easily open it again. To open it, double-click on HelloWorld.cpp in the Solution Explorer window.
Visual Studio Console Application Crack
If red squiggles appear under anything in the source code editor, check that your code matches the example in spelling, punctuation, and case. Case is significant in C++ code.
Visual Studio Console Application Code
Go back.